DevBG Presentation
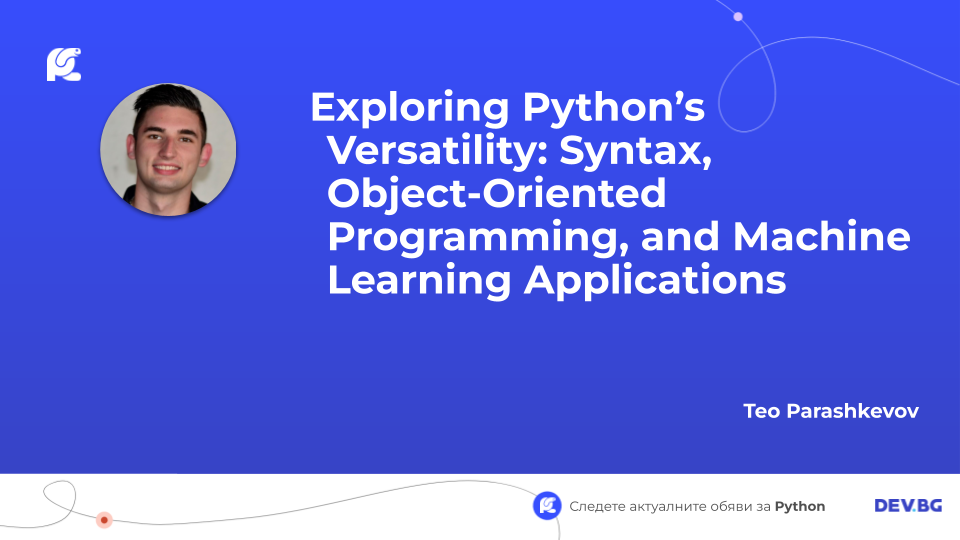
Introduction
This is a presentation on a comprehensive exploration of Python’s versatility, dissecting its syntax intricacies, delving into the Object-Oriented Programming (OOP), and concluding with its applications in the field of Machine Learning. I presented this topic on the 19 of September 2023 online on the biggest IT jobboard in Bulgaria - DevBG.
You can download the presentation as a PDF from here.
Why Python?
Python, an omnipresent language in the realm of programming, captivates us with its array of advantages over other languages. Its robust ecosystem, coupled with an intuitive syntax, fosters a conducive environment for seamless development. One of Python’s distinguishing features lies in its adeptness at resource management, augmenting efficiency in handling memory allocation and ensuring a smoother workflow. Furthermore, while speed might be perceived as a potential concern, Python showcases commendable performance when juxtaposed with its counterparts, substantiating its relevance across diverse domains.
Python’s allure transcends mere functionality; its virtues lie in its accessibility, fostering a paradigm of ease, readability, and rapid development. Unlike languages burdened with esoteric syntax, Python’s elegance eschews strange brackets, relying solely on indentation for logical separation. This simplicity, evident in file handling, starkly contrasts the verbosity of C. Consider the succinctness of Python’s four lines to open a file versus the twenty-eight lines entailed in C. Admittedly, the latter confers greater control over resources, yet Python’s concise syntax expedites development without sacrificing clarity.
The dynamic typing and high-level abstractions inherent in Python elevate it further. Common operations are encapsulated in intuitive constructs, hastening code production and comprehension. Moreover, Python’s cross-platform compatibility engenders code portability, a quintessential trait in today’s diverse technological landscape.
The expansive Python ecosystem, a hallmark of its prowess, encompasses machine learning, scientific frameworks, web development, and beyond. Its versatility extends far and wide, propelling innovation across myriad domains.
A comparative analysis unveils Python’s interpreted nature against C’s compiled architecture. Looping over 450 million numbers starkly illustrates this divergence. While C, being compiled, executes this task around 40 times faster than Python, the latter’s interpretive nature embodies flexibility and ease of prototyping, underscoring the trade-off between performance and development agility.
import sys
NUMBER = int(sys.argv[1])
s = 0
for i in range(NUMBER):
s += 1
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char **argv) {
int NUMBER, i, s;
NUMBER = atoi(argv[1]);
for (s = i = 0; i < NUMBER; ++i) {
s += 1;
}
return 0;
}
Syntax and Features
Python’s syntax is a tapestry of elegance and functionality, adorned with powerful features like context managers and decorators.
Context Managers:
At the heart of Python’s resource management lies context managers. These constructs, often employed with the “with” statement, encapsulate the acquisition and release of resources within a specific scope. Their primary advantage lies in ensuring resource cleanup and proper handling even in scenarios of exceptions or errors. For instance, when dealing with file operations, a context manager, such as the “open” function, automatically closes the file upon exiting the context. This mitigates the risk of resource leaks and enhances code reliability, allowing developers to focus on logic rather than intricate resource management.
Decorators:
Decorators, an equally compelling feature, enhance the flexibility and extensibility of functions or methods. These constructs empower developers to modify the behavior of functions without altering their original structure. By wrapping functions within other functions, decorators enable the addition of functionalities such as logging, authentication, or caching. This promotes code reusability and simplifies the implementation of cross-cutting concerns. Decorators serve as a testament to Python’s support for higher-order functions and metaprogramming, fostering cleaner and more modular codebases.
Object-Oriented Programming
Within Python’s realm of Object-Oriented Programming, the intricate interplay of classes, encapsulation, polymorphism, and inheritance lays the foundation for robust software design.
Classes and Abstractions:
Python, with its support for both normal and abstract classes, provides a versatile canvas for structuring code. These classes serve as blueprints for objects, encapsulating attributes and behaviors. Interfaces, although not strictly enforced in Python, enable developers to define contracts that classes can adhere to, fostering modularity and establishing a consistent structure within larger codebases.
Encapsulation, Polymorphism, and Inheritance:
Encapsulation, a cornerstone of OOP, shields data within classes, promoting data integrity and reducing complexity. Polymorphism, facilitated by Python’s dynamic typing, allows objects to exhibit different behaviors based on their context, fostering code flexibility and extensibility. Moreover, Python’s support for multiple inheritance, although controversial, offers a mechanism for combining functionalities from different classes, providing a powerful but nuanced tool that demands judicious use.
Dataclasses:
The advent of dataclasses represents a significant leap in simplifying the creation of structured data. These classes, introduced in Python 3.7, streamline the process of defining classes primarily meant for holding data. With minimal boilerplate code, dataclasses enhance readability and maintainability, catering to the modern developer’s need for concise yet expressive constructs.
Machine Learning
Python’s pervasive influence in Machine Learning stands as a testament to its adaptability and scalability across domains.
Keras and TensorFlow:
The amalgamation of Keras and TensorFlow embodies the cutting-edge of deep learning. Keras, known for its user-friendly interface, operates as a high-level neural networks API, while TensorFlow, with its robust ecosystem and computational efficiency, underpins the complex machinery of deep neural networks. Together, they democratize the creation of intricate models, accelerating the pace of innovation in artificial intelligence.
Scikit-learn:
Scikit-learn, a foundational library in Python, democratizes machine learning by offering a plethora of algorithms and tools. Its simplicity and ease of use make complex machine learning tasks accessible to a wider audience. From classification and regression to clustering and dimensionality reduction, Scikit-learn’s comprehensive suite empowers researchers and practitioners across various domains.